Awaiting on async method call in JS
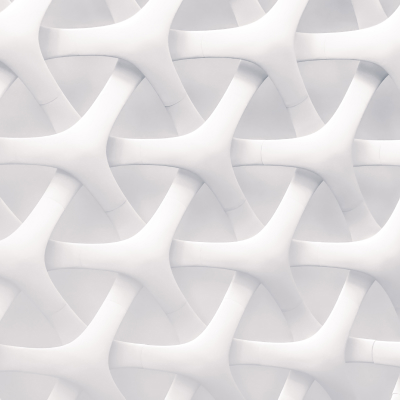
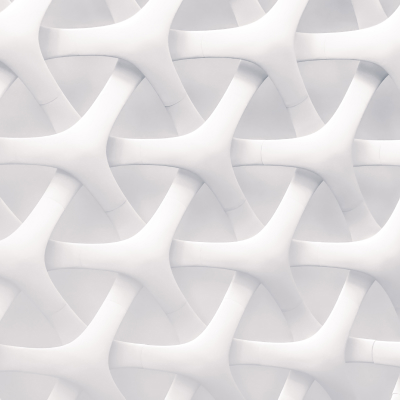
Have you ever been in a situation where you have spent hours together trying to debug as to why that database query is not awaited even after using the await
for an async method.
Lately, I ran into an issue where the database call was passed and the method run finished with the undefined in the database response even after using await. After spending hours trying to fix it, one article took me back top using a Promise
in javascript. Unfortunately this did not end the story as little was known to me that the two methods resolve
and reject
passed as params to the lambda call can be utilized to fetch the return response from that call like below.
export function getDataFromDb() {
var response = new Promise((resolve, reject) => {
....
// lines to fetch data from db
....
if(error) {
reject(error);
}
resolve(dbData);
})
return response
}
....
....
// make async call
var result = await getDataFromDb();
....
The resolve()
call actually returns the response from the database call or similar.
Promise
actually helps in awaiting the response from this or similar async calls and it also gives the benefit of reject
the response, if an error was found.
Another or the synchronous way of calling the promise method is by using the then
and/ or the catch
methods of the promise. The then
call's parameter should have the return value of the promise's method passed to the resolve
method and the catch
call's parameter is the parameter passed to the reject
method.
// make sync call
getDataFromDb()
.then(res => { console.log("Res from resolve is " + res) })
.catch(err => { console.error("Error from reject is " + err.msg) }
Having shown both the ways, it is for one to decide which way of calling the promise is better 😂 ...
How Promise works
Promise was apparently called or used as theneables
in JS back in the day, I am assuming it to be before ES6 or 2015.
To begin with let's understand Promise's signture as of today.
var Promise: PromiseConstructor
new <string>(executor: (resolve: (value: string | PromiseLike<string>) => void, reject: (reason?: any) => void) => void) => Promise<string>
Depending on the type
passed, resolve
must be called with the same type, in this case it is string, whereas the reject
can be of any
, may be new Error()
.
Promise takes in two methods as parameters. One to be called if the promise was completed successfully and the other to be called if not. The two paramters are called the resolve
and the reject
calls. The parameter passed to the resolve
method is the return value of the promise.
Why use Promise's
Promise's are more or less another way of using the try - catch
block if one looks closely in an asynchronous
way. Is it possible to run atry-catch
in an asynchronous
way? Using promises's again and again can help improve one's ability to understand their use cases better for sure.