C# vs Java programming comparison
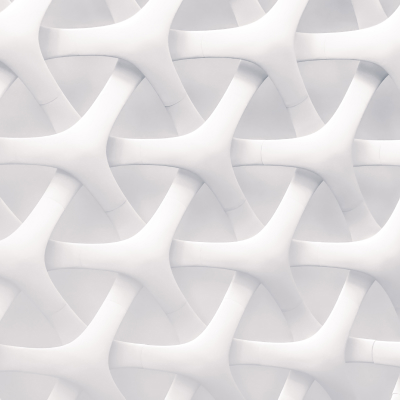
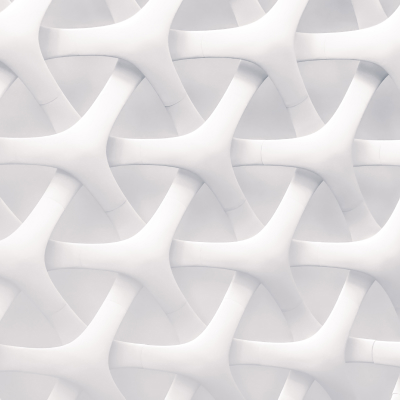
This guide is for those who like to learn Java and are from the C# background or vice - versa or similar who are pretty much versed in it. I have documented the aspects which needs to be grasped and is different from C#.
- First line of code can be say a Pgm.java file like below.
class Pgm
{
public static void main(String[] args)
{
System.out.println("Learning Java!);
}
}
-
The name of the file should be the same as the class inside. Main method is
main
here and string is String here. -
Inheritance -
-
Use
extends
for inheriting from another class. This can be from one class only. -
No virtual or override, instead methods of the same signatures are overridden.
-
Use
implements
to implement from an Interface and for multiple inheritance. -
If not implementing an interface, mark it as abstract. Only abstract classes can have abstract fields.
-
Classes -
-
Avoid modifiers to it, at least when learning. If using public it has to be in its own file.
-
Lambda methods - Similar to C#, use -> instead, like
Predicate<String> pred = s -> s.length() == 3;
and dopred.test("111")
which returnstrue
if matching the predicate. -
Compiling the source code.
javac Pgm.java
-
Running the program.
java Pgm
-
Packages - Like namespaces, supposed to be the name of the folder but no compilation errors will be issued. Use like
package abc.def.ghi;
When compiling, one may have to dojavac -d . Pgm.java
and when runningjava abc.def.ghi.Pgm
-
Statements -
-
All if, else, while and for loops are similar.
-
Switch cases can have multiple with comma separtions like
case SATURDAY, SUNDAY -> "week end";
-
Encapsulation - No properties here for classes. Create your own getter and setter individual methods.
-
Concurrent List - To make a list concurrent or thread safe, you can do
List newList = Collections.synchronizedList(eles);
-
Generics seem similar