Writing a method in different ways and others in JS
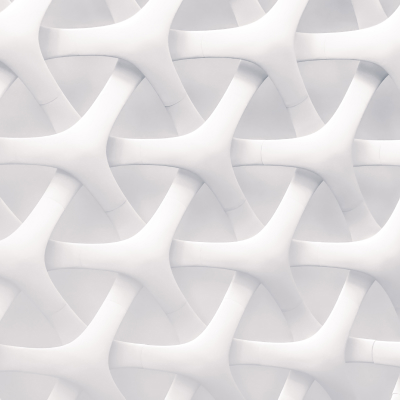
Ragavendra B.N.
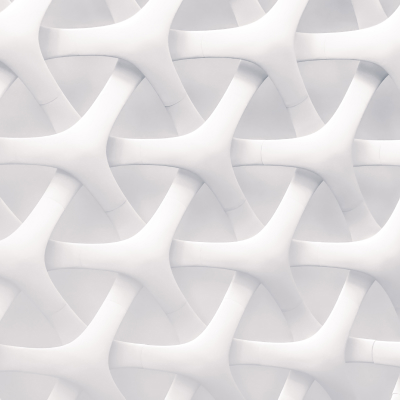
Ragavendra B.N.
Apparently there are at least three different ways of writing a method or a function in JS.
// Traditional way
function someVar(one, two) {
this.f = 'some str';
// #g = 3;
}
// JS way
const someVar = function (one, two) {
this.f = 'some str';
// #g = 3;
};
// Arrow function or lambdas in C#
const someVar = (one, two) => {
this.f = 'some str';
// #g = 3;
};
Apparently for OOP, back in the day, JS did not have classes and a method itself was used as a class like below.
function someVar(one, two) {
this.f = 'some str';
// #g = 3;
}
const frd = new someVar(1, 6);
console.info("someVar is " + frd.f);
An object can have a property or a method like below.
const newVar = {
frd: 'some str here',
sfr() {
console.info('This is in sfr');
},
};
One object's methods or properties can be copied or updated to another object aka inheritance or extending the object itself using object's prototype
and assign .
function someVar(one, two) {
this.f = 'some str';
// #g = 3;
this.bgt = () => {
console.info('In bgt now');
return 1;
};
}
Object.assign(someVar.prototype, newVar);
newVar.sfr();
console.log('Afre adding ' + new someVar(1, 3).frd);
Suppose one has imported a JS library from unpkg.com
. The library does not have a new functionality, it can be easily added or extended this way.