Interface versus Abstract class
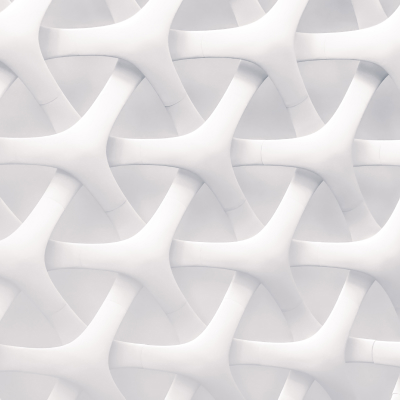
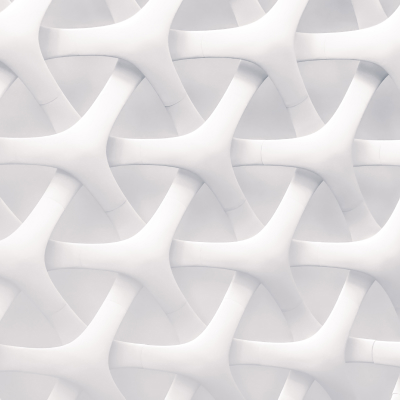
One of the common questions one can face when learning Object oriented programming language is when to use abstract classes and when to use interfaces. Having heard about this question in common interview questions online also sparks curiosity, atleast to me and it no doubt lingered for atleast quite a number of years until today. Also, interesting to note that if you learnt about abstract class and interfaces and this question became obvious to you is a great sign that your headed in the right direction in your programming career.
Now lets get to our discussion without further adieu. Let me first put a reasonable example for the explanation to follow realated to our subject.
abstract class Plant
{
public int id;
public String leafShape;
public abstract void BearFruit();
public abstract void BearLeaf();
public void Grow(){
BearLeaf();
BearLeaf();
BearFruit();
}
}
class Parsley extends Plant{
public void BearFruit() {
System.out.println("Bearing fruit.");
}
@Override
public void BearLeaf() {
System.out.println("Bearing leaf.");
}
}
The sample above has an abstract class Plant
which is extended by the class Parsley
. Note that there are couple of abstract methods as well like the BearLeaf
and the BearFruit
which are overridden in the Parsley class.
When one extends an abstract class, they are actually saying that the extended class is a type of the parent class. Parsley is a Plant, whereas one implements an interface, say for example an InfoBox interface, one is actually implementing the InfoBox and Parsley is not an InfoBox. This is the main reason behind the explanation as to why abstract class and interfaces both co - exist.
The second main difference is that only one class can extend an abstract class, whereas multiple interfaces can be implemented by a class.
Lastly the final reasonable diffirence is that, all the method(s) ha(s)ve to be declared in the abstract class, but not in the interface.
In summary, we learnt the reasons as to when to use an abstract class and when an interface. I hope this article has given enough insight into the abstract class and the interface. Please feel free to subscribe to my newsletter or my blog for further articles