Mocking Next request and responses
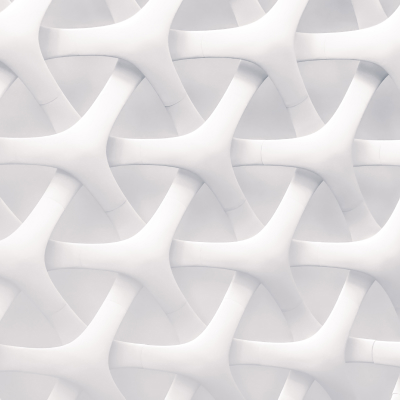
Ragavendra B.N.
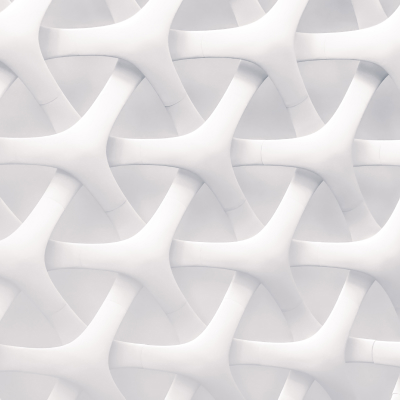
Ragavendra B.N.
Writing unit tests for NextJS is one area that needs some light to be shed upon as the framework has been gaining ground lately especially due to its static site generation ( SSG ), periodic SSG and using react as front end. node-mocks-http is one such library which claims that NextApiRequest
and NextApiResponse
are inherited from Node's own request and response.
Suppose your api looks like below.
// pages/api/hello.ts
import { NextApiRequest, NextApiResponse } from 'next';
export default async function hello(req: NextApiRequest, res: NextApiResponse<Resp>) {
return new Promise<void>( (resolve, reject) => {
switch (req.method) {
case 'GET':
res.status(200).json({ message: "Hello call resp", code: 200 });
break;
default: {
const resp = `Method ${req.method} Not Allowed`;
res.setHeader('Allow', ['GET']);
res.status(405).send({ message: resp, code: 400 });
}
}
resolve();
})
}
You can have the test for it as below.
// pages/api/hello.test.ts
/* eslint-env jest */
import { describe, expect, test } from '@jest/globals';
import { NextApiRequest, NextApiResponse } from 'next/types';
import httpMocks from 'node-mocks-http';
import hello from './hello';
require('iconv-lite').encodingExists('foo');
describe('gtfsTripUpdate api', () => {
test('hello positive', () => {
const mockExpressRequest = httpMocks.createRequest<NextApiRequest>({
method: 'GET',
});
const mockExpressResponse = httpMocks.createResponse<NextApiResponse>();
hello(mockExpressRequest, mockExpressResponse).then(() => {
expect(mockExpressResponse._getStatusCode()).toBe(200);
expect(mockExpressResponse._getJSONData().message).toEqual("Hello call resp");
});
});
});
I am using then
, one can use the await
and async
as well.